CoreStack External APIs
Learn the basics of CoreStack's APIs and how you can get started using them.
Overview
CoreStack offers a wide range of REST APIs to integrate with your product or external services/tools programmatically. With REST API support it's possible to integrate with almost all of the programming languages, while the integration varies from one language to the other.
CoreStack's API guide not only provides information about the REST APIs available in cURL format but also provides the code snippets for widely-used programming languages, such as Java, Python, JavaScript, PHP, and so on.
Before drilling into our API reference, it's important to understand some key fundamentals.
CoreStack Organization – Tenant Structure
CoreStack out of the box supports multi-tenancy. In enterprises and IT organizations, there are bound to be multiple departments. Associates and resources under each department often need to be isolated from each other. This isolation is not only needed for financial accounting but also for security and management purposes. Associates can have access to multiple departments, but their permission levels will likely vary between departments.
In an enterprise, some common departments include Operations, Finance, and R&D -- these can also be considered as tenants in CoreStack.
The below diagram shows a sample mapping for a similar setup:
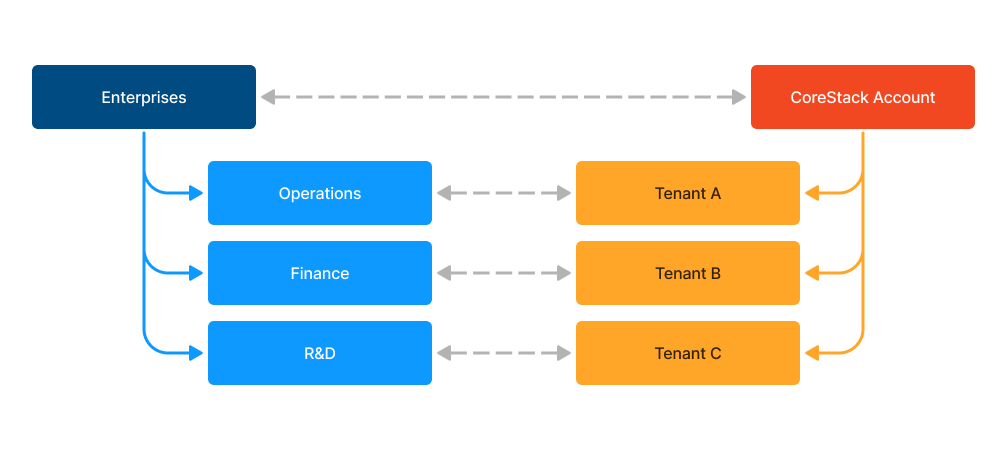
Model 1: Mapping Enterprise and CoreStack Structure
In an IT organization, some common teams include QA, Engineering, and DevOps -- these can also be considered as tenants in CoreStack.
The below diagram shows a sample mapping for a similar setup:
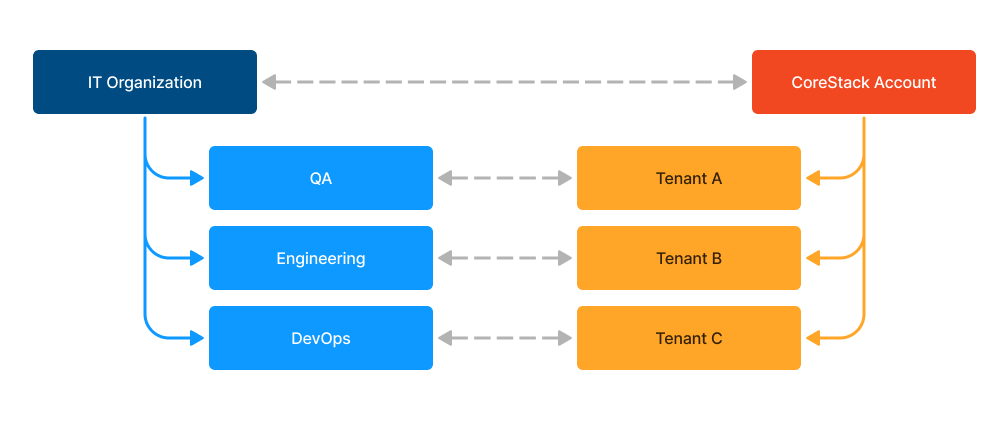
Model 2: Mapping IT Organization and CoreStack Structure
For more detailed information about Accounts, Tenants, Users, and Roles, please refer to our other user guides.
CoreStack API Modules
The CoreStack API is organized around the following modules. Please note that this is very similar to the CoreStack cloud governance pillars around OSCAR, compliance, and self-service.
S.No. | Module Name | Module Description |
---|---|---|
1 | Authorization | These APIs provide a secure authorization mechanism to access the CoreStack APIs. |
2 | Identity | These APIs will provide you with the ability to manage CoreStack account-related identity and access information such as tenants, users, roles and permissions, passwords, etc. |
3 | Guardrails | These APIs will help you to create, manage, and execute the guardrail policies using CoreStack on your cloud accounts. The policies can be used to assess, audit, and evaluate the configurations of your cloud resources so that those resources stay compliant with your corporate standards and service level agreements (SLAs). |
4 | Account Governance | These APIs allow you to manage and set up assessments of your cloud accounts against CoreStack cloud governance pillars. |
5 | Operations | These APIs will provide you with the ability to operate and manage your cloud resources efficiently using various features such as automation, monitoring, notifications, and activity tracking. |
6 | Security | These APIs will provide you with the ability to manage the security governance of your cloud accounts as well as identify security vulnerabilities and threats, and resolve them. |
7 | Cost | These APIs will provide you with the ability to create a customized controlling mechanism that can control your cloud expenses within budgets and reduce cloud waste by continually discovering and eliminating inefficient resources. |
8 | Access | These APIs will provide you with the ability to configure secure access of resources in your cloud environment and protect users’ data and assets from unauthorized access. |
9 | Resource | These APIs will provide you with the ability to define, enforce, and track the resource naming and tagging standards, sizing, and their usage by regions. It also enables you to follow consistent and standard practices pertaining to resource deployment, management, and reporting. |
10 | Compliance | These APIs will provide you with the ability to assess your cloud environment for its compliance status against standards and regulations that are relevant to your organization – ISO, NIST, HIPAA, PCI, CIS, FedRAMP, AWS Well-Architected framework, and custom standards. |
11 | Self Service | These APIs will provide you with the ability to configure a simplified self-service cloud consumption model for end users that is tied to approval workflows. It enables you to automate repetitive tasks and focus on key deliverables. |
Authentication and Authorization
Introduction
To access the CoreStack API, you will need an auth token and username.
The auth token is a short-lived token that provides authorization to perform API operations. An auth token can be generated using the Authorization API by providing the Access Key and Secret Key.
The Access Key and Secret Key are the access credentials you would need to generate the authorization token. The AuthToken API is used to generate an Auth Token by providing these access credentials.
Once the user is terminated or suspended in the CoreStack portal, the associated user's "Access Key" and "Secret Key" will no longer be working. If the user authentication is enabled using SSO, the user will be created in the CoreStack portal but the authentication will be using the user organization LDAP. Once the user is terminated or suspended in the organization LDAP, the user can longer authenticate to access the CoreStack portal. In this case, the respective user's "Access Key" needs to be deleted in the CoreStack portal.
All other CoreStack API calls require a Username and Auth-token. A few API calls, in addition, may require TenantID and/or Account ID as well. All these values are available in the authorization API response.
A CoreStack account can have multiple tenants, which is why providing a tenant ID is mandatory in order to perform API operations under the corresponding tenant.
Refer to the API guide for the detailed information about AuthToken (Authentication) API.
Authentication Headers
The following authentication headers must be available as part of all API requests:
Header | Value |
---|---|
Content-Type | application/json |
X-Auth-User | Username of the tenant |
X-Auth-Token | Token generated as part of AuthToken API |
Note:
X-Auth-User and X-Auth-Token are required for all API operations -- except the Authentication API.
Sample API Request with Authentication Headers
The following example demonstrates how to use authentication headers and retrieve the list of all tenants within a CoreStack Account.
curl -X GET -H “X-Auth-Token: [[Access Token]]” -H “X-Auth-User: [[username]]” https://api-discover.corestack.io/v1/tenants
curl -X GET -H “X-Auth-Token: abcedefghijklmno34567890” -H “X-Auth-User: cs_user” https://api-discover.corestack.io/v1/tenants
How to get the Access Key and Secret Key
In order to access CoreStack APIs, a user must have an Access Key and Secret Key.
Follow the steps provided below to generate an Access Key and Secret Key:
- Login to CoreStack as ops_admin.
- Navigate to the Users Page:
a. Click the settings icon in the top-right.
b. Click Users from the menu.
c. The Users page will be displayed along with the list of users available under the CoreStack Account. - Click ‘+‘ icon to add a new user.
- Fill in the necessary details and provide a valid email address.
- The role and tenant access determines the access control for the user and the APIs they can access.
- Select the checkbox “Generate API Access” in the bottom-right.
- Click Create User.
- The user will receive an activation email to create a password for the newly created user account.
Once it is activated, the user account will be successfully created. The user also receives an email with the API URL, Access Key, and Secret Key.
Regenerate Access/Secret Keys
Perform the following steps to regenerate the Access Key and/or Secret Key:
- Login to CoreStack as the user that has the privilege to manage users (e.g., ops_admin).
- Navigate to Users Page:
a. Click the settings icon in the top-right.
b. Click Users from the menu.
c. The Users page will be displayed along with the list of users available under the CoreStack Account. - Select the user for whom the API keys need to be regenerated.
- Expand the section API Access in the right pane.
- This section will have the Access Key and Valid Till information along with a “REGENERATE KEY” button.
- Clicking on the “REGENERATE KEY” button will overwrite the existing key and create a new key. The old keys will become invalid after regenerating.
- The user will receive an email with the regenerated Access Key and Secret Key.
How to Retrieve the Authorization Token
Once you have the access credentials, you can create an authorization token using the Authorization API.
The following code demonstrates how to retrieve authorization token using AuthToken API.
Use the following AuthToken API to generate an authorization token:
curl -X POST -d {"access_key":"[[AccessKey}}", "secret_key":"SecretKey"} -H "Content-Type: application/json" https://api-discover.corestack.io/v1/auth/tokens
curl -X POST -d {"access_key":"a1b2c3d4e5f6g7h8i9j", "secret_key":"abcdefghijk1234567890"} -H "Content-Type: application/json" https://api-discover.corestack.io/v1/auth/tokens
How to Use the Authorization Token
The following example demonstrates how to use the authentication token generated using the AuthToken API and access CoreStack's external APIs.
Construct an API request similar to the one explained below for requesting API operation. The following example explains how to retrieve the list of all tenants within a CoreStack Account:
curl -X GET -H “X-Auth-Token: [[Access Token]]” -H “X-Auth-User: [[username]]” https://api-discover.corestack.io/v1/tenants
curl -X GET -H “X-Auth-Token: abcedefghijklmno34567890” -H “X-Auth-User: cs_user” https://api-discover.corestack.io/v1/tenants
Note:
All the CoreStack APIs can be tried virtually in the Developer Portal.
HTTP Verbs
The following table contains the REST API HTTP Verbs supported by CoreStack.
Verb | Operation |
---|---|
GET | To lList all resources or Get a specific resource. Request Body is not supported. |
PUT | To Update properties of existing resources. Also used to perform specific actions on an existing resource. |
POST | To Create new resource and in some cases used for fetching resources with complex parameters which cannot be accommodated in GET method. |
DELETE | To Delete one or more existing resources. |
Response Codes
The following table provides a list of response codes that can be expected in response to an API request, and describes the result of an API operation.
Response Code | Description |
---|---|
200 – Ok | The operation was successful, and a response has been returned (GET and PUT requests). |
201 – Created | The operation was successful, the posted entity has been created and requested information is returned in the response-body (POST request). |
204 – No Content | The operation was successful, the requested entity has been deleted and therefore there is no response-body returned (DELETE request). |
401 – Unauthorized | The operation failed. The operation requires Authentication headers to be set. If this was present in the request, there are chances that supplied credentials are not valid or the user is not authorized to perform this operation. X-Auth-User & X-Auth-Token headers should have valid credentials. |
403 – Forbidden | The operation is forbidden and should not be re-attempted. This does not imply an issue with authentication, it’s an operation that is not allowed. Example: deleting a task that is part of a running process is not allowed and will never be allowed, regardless of the user or process/task state. |
404 – Not Found | The operation failed. The requested resource was not found. |
405 – Method Not Allowed | The operation failed. The HTTP method used is not allowed for this resource. Example: trying to update (PUT) a deployment-resource will result in a 405 status. |
409 – Conflict | The operation failed. The operation causes an update of a resource that has been updated by another operation, which makes the update no longer valid. Can also indicate a resource that is being created in a collection where a resource with that identifier already exists. |
500 – Internal Server Error | The operation failed. An unexpected exception occurred while executing the operation. The response-body contains needed details about the error. |
Supported Data Types – URL Query Parameters
CoreStack supports widely used data types as part of the URL query parameters, as shown in the table below:
Type | Format |
---|---|
String | Plain text parameters. Can contain special characters, numerals and alphabets with proper URL encoding. |
Integer | Parameter representing an integer value. Can only contain numeric non-decimal values, between -2.147.483.648 and 2.147.483.647 |
Long | Parameter representing a long value. Can only contain numeric non-decimal values, between -9.223.372.036.854.775.808 and 9.223.372.036.854.775.807 |
Boolean | Parameter representing a Boolean value. Can be either true or false. Any other values other than these two, will be considered as a string input throwing 405 – Bad request response. |
Date | Parameter representing a date value. Use the ISO-8601 date-format (see ISO-8601 on Wikipedia) with both time and date values (e.g., 2013-04-03T23:45Z). |
Supported Data Types – Request/Response JSON Data Types
CoreStack supports widely used data types as part of the request/response body, as shown in the table below:
Type | Format |
---|---|
String | Plain text parameters. |
Integer | Parameter representing an integer value. Can only contain numeric non-decimal values, between -2.147.483.648 and 2.147.483.647 |
Long | Parameter representing a long value. Can only contain numeric non-decimal values, between -9.223.372.036.854.775.808 and 9.223.372.036.854.775.807 |
Boolean | Parameter representing a Boolean value. Can be either true or false. Any other values other than these two, will be considered as a string input throwing 405 – Bad request response. |
Date | Parameter representing a date value, using a JSON text. Use the ISO-8601 date-format (see ISO-8601 on Wikipedia) using both time and date-components (e.g., “2013-04- 03T23:45Z”). |
Other Information
Pagination
Pagination applies to only LIST actions such as ListTenants, ListUsers and so on.
Query Parameter Usage
'Limit' in query parameter is used to limit the number of records in the response, whereas 'page' in query parameter is used to provide the page number.
http://<list_api_endpoint>?limit=25&page=2
Actions not supported through API
The following actions are not supported by CoreStack through APIs.
- CloudAccount API actions for Azure
Note:
To view the list of APIs, click here.
Updated over 1 year ago